Object Detection using SSD-MobileNetv3
import cv2
import matplotlib.pyplot as plt
classLabels = []
file_name = 'Labels.txt'
with open(file_name,'rt') as fpt:
classLabels = fpt.read().rstrip('\n').split('\n')
print(classLabels) ## Class labels

config_file = 'ssd_mobilenet_v3_large_coco_2020_01_14.pbtxt'
frozen_model = 'frozen_inference_graph.pb'
Reading the Model in Opencv
#model = cv2.dnn.readNetFromTensorflow(frozen_model,config_file)
model = cv2.dnn_DetectionModel(frozen_model,config_file)
model.setInputSize(320,320)
model.setInputScale(1.0/127.5) ## 255/2 = 127.5
model.setInputMean((127.5,127.5,127.5)) ##
model.setInputSwapRB(True)
<dnn_Model 000001BDE265BA20>
#model.setInputSize(300,300)
#model.setInputScale(1.0/127.5) ## 255/2 = 127.5
#model.setInputMean((255,255,255)) ##
#plt.imshow(cv2.cvtColor(frame, cv2.COLOR_BGR2RGB))
img = cv2.imread('man-bmw.png')
img = cv2.imread('man_tuxedo_car_style_27329_1920x1080.jpg')
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
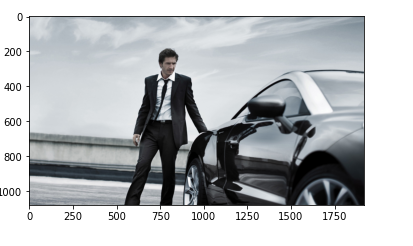
ClassIndex, confidece, bbox = model.detect(img,confThreshold=0.5)
print(ClassIndex)
[[1]
[3]]
type(ClassIndex)
numpy.ndarray
id =(ClassIndex-1).flatten()
print(id)
[0 2]
type(id.flatten())
classLabels[ClassIndex.item()-1]
font_scale = 3
font = cv2.FONT_HERSHEY_PLAIN
for ClassInd, conf, boxes in zip(ClassIndex.flatten(), confidece.flatten(), bbox):
#cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2)
#cv2.putText(img, text, (text_offset_x, text_offset_y), font, fontScale=font_scale, color=(0, 0, 0), thickness=1)
cv2.rectangle(img,boxes,(255, 0, 0), 2 )
cv2.putText(img,classLabels[ClassInd-1],(boxes[0]+10,boxes[1]+40), font, fontScale=font_scale,color=(0, 255, 0), thickness=3 )
boxes[1]
294
import matplotlib.pyplot as plt
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
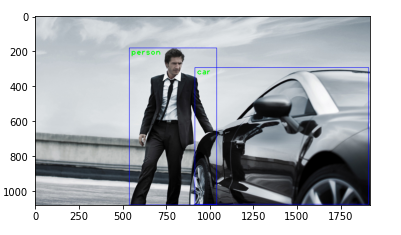
print(len(classLabels))
80
Video Reading and Applying Object Detection
cap = cv2.VideoCapture("Record_2020_10_16_02_31_46_801.mp4")
# Check if the webcam is opened correctly
if not cap.isOpened():
cap = cv2.VideoCapture(0)
if not cap.isOpened():
raise IOError("Cannot open webcam")
font_scale = 3
font = cv2.FONT_HERSHEY_PLAIN
while True:
ret,frame = cap.read()
ClassIndex, confidece, bbox = model.detect(frame,confThreshold=0.55)
print(ClassIndex)
if (len(ClassIndex)!=0):
for ClassInd, conf, boxes in zip(ClassIndex.flatten(), confidece.flatten(), bbox):
if (ClassInd<=80):
cv2.rectangle(frame,boxes,(255, 0, 0), 2 )
cv2.putText(frame,classLabels[ClassInd-10],(boxes[0]+10,boxes[1]+40), font, fontScale=font_scale,color=(0, 255, 0), thickness=3 )
cv2.imshow('Object Detection Tutorial',frame)
if cv2.waitKey(2) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
For Webcame Demo
cap = cv2.VideoCapture(1)
# Check if the webcam is opened correctly
if not cap.isOpened():
cap = cv2.VideoCapture(0)
if not cap.isOpened():
raise IOError("Cannot open webcam")
font_scale = 3
font = cv2.FONT_HERSHEY_PLAIN
while True:
ret,frame = cap.read()
ClassIndex, confidece, bbox = model.detect(frame,confThreshold=0.55)
print(ClassIndex)
if (len(ClassIndex)!=0):
for ClassInd, conf, boxes in zip(ClassIndex.flatten(), confidece.flatten(), bbox):
if (ClassInd<=80):
cv2.rectangle(frame,boxes,(255, 0, 0), 2 )
cv2.putText(frame,classLabels[ClassInd-10],(boxes[0]+10,boxes[1]+40), font, fontScale=font_scale,color=(0, 255, 0), thickness=3 )
cv2.imshow('Object Detection Tutorial',frame)
if cv2.waitKey(2) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()