import pytesseract
import cv2 # pip install opencv-python
import matplotlib.pyplot as plt ## pip install matplotlib
For Configuration
pytesseract.pytesseract.tesseract_cmd = r'C:\\Program Files\\Tesseract-OCR\\tesseract.exe'
img = cv2.imread('Demo.png')
plt.imshow(img)
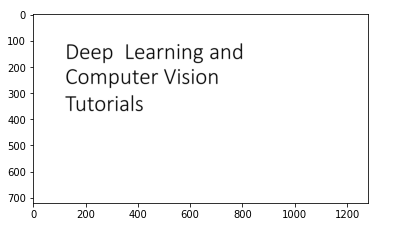
img2char = pytesseract.image_to_string(img)
print(img2char)
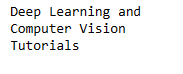
imgbox = pytesseract.image_to_boxes(img)
type(imgbox)
str
print(imgbox)
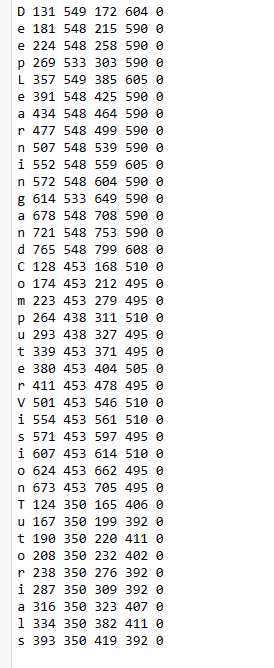
imgH, imgW,_ = img.shape
img.shape
(720, 1280, 3)
for boxes in imgbox.splitlines():
boxes = boxes.split(' ')
x,y,w, h = int(boxes[1]),int(boxes[2]),int(boxes[3]),int(boxes[4])
cv2.rectangle(img, (x,imgH-y), (w,imgH-h), (0,0,255),3)
plt.imshow(img) ## by default cv2=> BGR
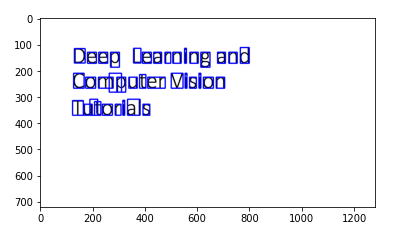
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
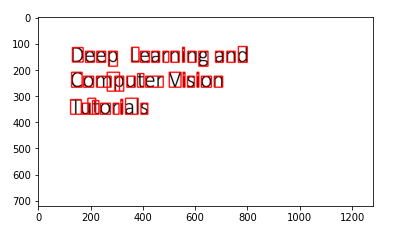
Video Demo
#import cv2 ### pip install opencv-python
## pip install opencv-contrib-python fullpackage
#import numpy as np
font_scale = 1.5
font = cv2.FONT_HERSHEY_PLAIN
#cap = cv2.VideoCapture(1)
cap = cv2.VideoCapture("Record_2020_10_23_23_37_17_468.mp4")
#cap.set(cv2.CAP_PROP_FPS, 170)
if not cap.isOpened():
cap = cv2.VideoCapture(0)
if not cap.isOpened():
raise IOError("Cannot open video")
cntr =0;
while True:
ret,frame = cap.read()
cntr= cntr+1;
if ((cntr%20)==0):
imgH, imgW,_ = frame.shape
x1,y1,w1,h1 = 0,0,imgH,imgW
imgchar = pytesseract.image_to_string(frame)
imgboxes = pytesseract.image_to_boxes(frame)
for boxes in imgboxes.splitlines():
boxes= boxes.split(' ')
x,y,w,h= int(boxes[1]),int(boxes[2]),int(boxes[3]),int(boxes[4])
cv2.rectangle(frame, (x, imgH-y),(w,imgH-h),(0,0,255),3)
#cv2.rectangle(frame, (x1, x1), (x1 + w1, y1 + h1), (0,0,0), -1)
# Add text
cv2.putText(frame, imgchar, (x1 + int(w1/50),y1 + int(h1/50)), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0), 2)
font = cv2.FONT_HERSHEY_SIMPLEX
#gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
#print(faceCascade.empty())
#faces = faceCascade.detectMultiScale(gray,1.1,4)
# Draw a rectangle around the faces
#for(x, y, w, h) in faces:
# cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
# Use putText() method for
# inserting text on video
cv2.imshow('Text Detection Tutorial',frame)
if cv2.waitKey(2) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
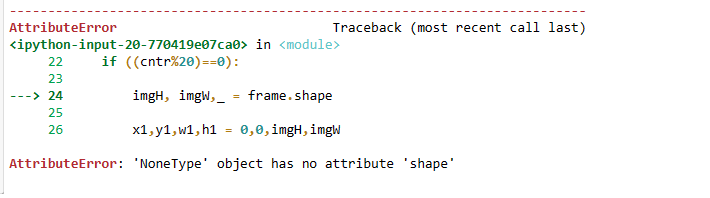
Webcame
#import cv2 ### pip install opencv-python
## pip install opencv-contrib-python fullpackage
#import numpy as np
font_scale = 1.5
font = cv2.FONT_HERSHEY_PLAIN
cap = cv2.VideoCapture(1)
#cap = cv2.VideoCapture("Record_2020_10_23_23_37_17_468.mp4")
#cap.set(cv2.CAP_PROP_FPS, 170)
if not cap.isOpened():
cap = cv2.VideoCapture(0)
if not cap.isOpened():
raise IOError("Cannot open video")
cntr =0;
while True:
ret,frame = cap.read()
cntr= cntr+1;
if ((cntr%20)==0):
imgH, imgW,_ = frame.shape
x1,y1,w1,h1 = 0,0,imgH,imgW
imgchar = pytesseract.image_to_string(frame)
imgboxes = pytesseract.image_to_boxes(frame)
for boxes in imgboxes.splitlines():
boxes= boxes.split(' ')
x,y,w,h= int(boxes[1]),int(boxes[2]),int(boxes[3]),int(boxes[4])
cv2.rectangle(frame, (x, imgH-y),(w,imgH-h),(0,0,255),3)
#cv2.rectangle(frame, (x1, x1), (x1 + w1, y1 + h1), (0,0,0), -1)
# Add text
cv2.putText(frame, imgchar, (x1 + int(w1/50),y1 + int(h1/50)), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (255,0,0), 2)
font = cv2.FONT_HERSHEY_SIMPLEX
#gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
#print(faceCascade.empty())
#faces = faceCascade.detectMultiScale(gray,1.1,4)
# Draw a rectangle around the faces
#for(x, y, w, h) in faces:
# cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
# Use putText() method for
# inserting text on video
cv2.imshow('Text Detection Tutorial',frame)
if cv2.waitKey(2) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()